Created by N Padmavathi on Jan 08, 2024
Extension ID
com.castsoftware.dotnet.odata
What's new?
See OData Client for .NET - 1.0 - Release Notes.
Description
This extension provides support for OData client libraries when used inside .NET source code.
In what situation should you install this extension?
If your .NET application source code uses the odata client libraries to consume OData services, you should install this extension.
Support
Libraries supported
APIs supported
Click a category to expand |
---|
OData v4 DataServiceQuery APIs - Microsoft.OData.Client.DataServiceQuery.Execute
- Microsoft.OData.Client.DataServiceQuery.ExecuteAsync
OData v4 DataServiceContext APIs - Microsoft.OData.Client.DataServiceContext.AddObject
- Microsoft.OData.Client.DataServiceContext.CreateQuery
- Microsoft.OData.Client.DataServiceContext.DeleteObject
- Microsoft.OData.Client.DataServiceContext.Execute
- Microsoft.OData.Client.DataServiceContext.ExecuteBatch
- Microsoft.OData.Client.DataServiceContext.UpdateObject
|
OData v1-v3 DataServiceQuery APIs - System.Data.Services.Client.DataServiceQuery.AddQueryOption
- System.Data.Services.Client.DataServiceQuery.Execute
- System.Data.Services.Client.DataServiceQuery.Expand
- System.Data.Services.Client.DataServiceQuery.IncludeTotalCount
OData v1-v3 DataServiceContext APIs - System.Data.Services.Client.DataServiceContext.AddObject
- System.Data.Services.Client.DataServiceContext.CreateQuery
- System.Data.Services.Client.DataServiceContext.DeleteObject
- System.Data.Services.Client.DataServiceContext.Execute
- System.Data.Services.Client.DataServiceContext.UpdateObject
|
Simple.OData APIs - Simple.OData.Client.IBoundClient.FindEntriesAsync
- Simple.OData.Client.IBoundClient.InsertEntriesAsync
- Simple.OData.Client.IBoundClient.UpdateEntriesAsync
- Simple.OData.Client.IBoundClient.DeleteEntriesAsync
- Simple.OData.Client.IBoundClient.FindEntryAsync
- Simple.OData.Client.IBoundClient.InsertEntryAsync
- Simple.OData.Client.IBoundClient.UpdateEntryAsync
- Simple.OData.Client.IBoundClient.DeleteEntryAsync
|
|
Function Point, Quality and Sizing support
This extension provides the following support:
Function Points (transactions) | Quality and Sizing |
---|
 |  |
AIP Core compatibility
AIP Core release | Supported |
---|
8.3.x |  |
Prerequisites
 | An installation of any compatible release of AIP Core (see table above) |
What source code is needed by our analyzer?
For applications using Microsoft libraries, the OData extension requires the client proxy file, service reference file (.xml, .edmx etc.) generated by the code generation tool to function correctly.
Dependencies with other extensions
Some CAST extensions require the presence of other CAST extensions in order to function correctly. The OData extension requires the following CAST extensions to be installed:
Download and installation instructions
For .NET applications using OData client libraries, the extension will be automatically installed by CAST Console. This is in place since October 2023.
For upgrade, if the Extension Strategy is not set to Auto update, you can manually install the extension using the Application - Extensions interface.
What results can you expect?
Objects
The following objects are displayed in CAST Enlighten:
Icon | Description |
---|
| OData Client HttpRequest Get Service |
| OData Client HttpRequest Post Service |
| OData Client HttpRequest Put Service |
| OData Client HttpRequest Patch Service |
| OData Client HttpRequest Delete Service |
Links
Link Type | Source and Destination |
---|
callLink | Link from C# method making API call to service object (eg. OData Client HttpRequest Get Service) |
Examples
Insert Operation
Click here to expand...
using System;
using System.Diagnostics;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Data.Services.Client;
using WpfApplication1.ServiceReference1;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void Window1_Loaded(object sender, RoutedEventArgs e)
{
try
{
var svcUri = "http://localhost:52027/WcfDataService1.svc";
var context = new castEntities(new Uri(svcUri));
WpfApplication1.ServiceReference1.Table newProduct = WpfApplication1.ServiceReference1.Table.CreateTable(4);
newProduct.Name = "Rian";
context.AddObject("Tables", newProduct);
context.SaveChanges();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}
private void buttonClose_Click(object sender, RoutedEventArgs e)
{
this.Close();
}
}
}
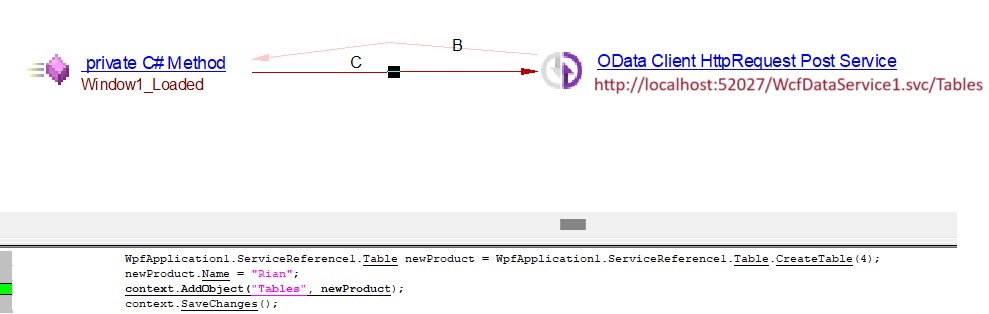
Select Operation
Click here to expand...
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using WebApplication2.Models;
namespace odata_client_new
{
class Program
{
static void Main(string[] args)
{
ListProduct().Wait();
}
static async Task ListProduct()
{
var serviceRoot = "http://localhost:64700/odata";
var context = new Default.Container(new Uri(serviceRoot));
IEnumerable<Product> products = await context.Products.ExecuteAsync();
foreach (var product in products)
{
Console.WriteLine("{0} --- {1}", product.ID, product.Name);
}
}
}
}
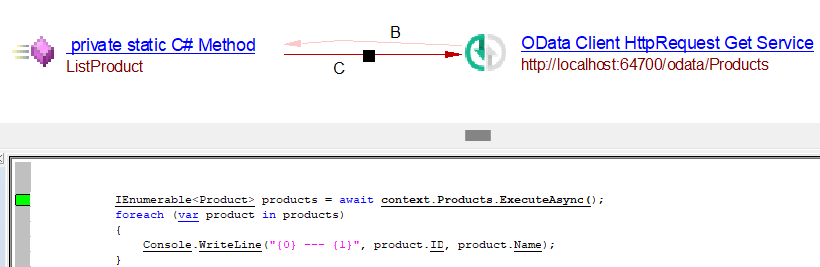
Update Operation
Click here to expand...
using System;
using System.Diagnostics;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Data.Services.Client;
using WpfApplication1.ServiceReference1;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void Window1_Loaded(object sender, RoutedEventArgs e)
{
try
{
Uri svcUri = new Uri("http://localhost:52027/WcfDataService1.svc");
context = new castEntities(svcUri);
var data_to_change = (from table in context.Tables
where table.Name == "Sam"
select table).Single();
data_to_change.Name = "Wiley";
context.UpdateObject(data_to_change);
context.SaveChanges();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}
private void buttonClose_Click(object sender, RoutedEventArgs e)
{
this.Close();
}
}
}
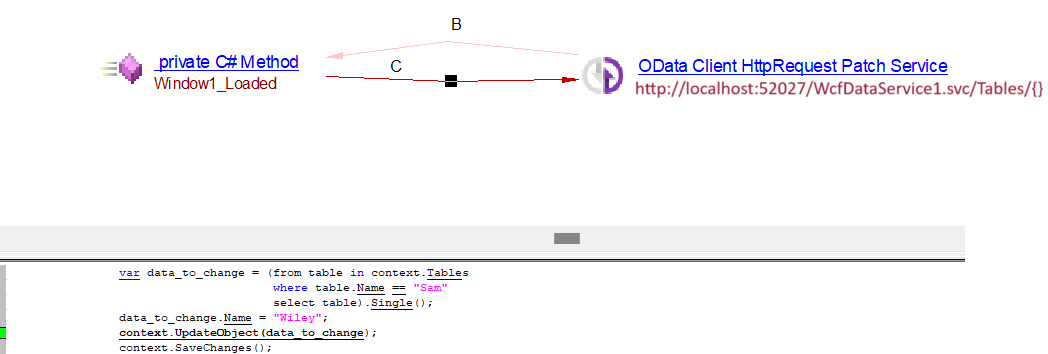
Delete Operation
Click here to expand...
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using WebApplication2.Models;
namespace odata_client_new
{
class Program
{
static void Main(string[] args)
{
DeleteCustomer().Wait();
}
static async Task DeleteCustomer()
{
var serviceRoot = "http://localhost:64700/odata";
var context = new Default.Container(new Uri(serviceRoot));
var customer = await context.Customers.ByKey(new Dictionary<string, object>() { { "ID", 002 } }).GetValue();
Console.WriteLine(customer.Name);
context.DeleteObject(customer);
context.SaveChanges();
}
}
}
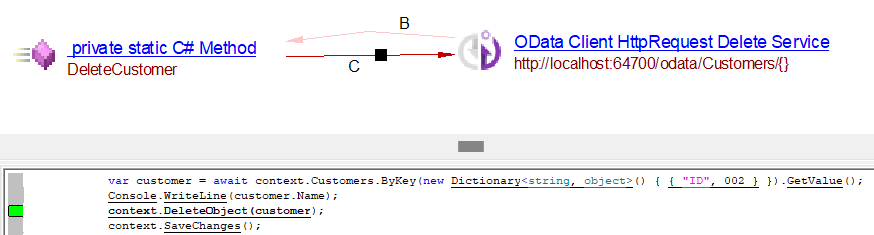
Universal Linker is responsible for linking service object (eg. OData Client HttpRequest Get Service) to any matching operation objects. (Operation objects are generated by the analysis of server code. Refer dotnetweb documentation.)
Limitations